The following 4 steps will help you create a spring boot application in 30 minutes or less.
Step 1 :
Go to start.spring.oi (Spring Initializer) and select Maven as build tool. Select Java as language with Spring boot latest version (avoid taking snapshot version)
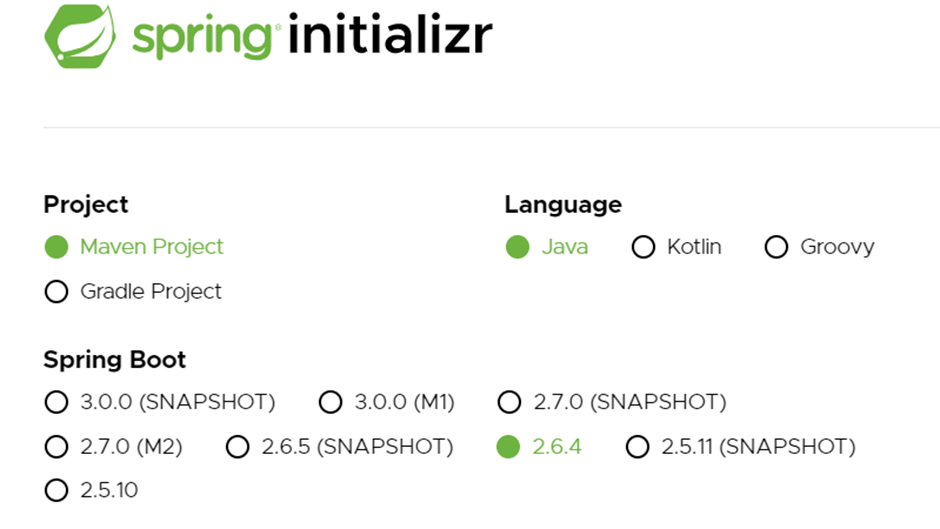
Step 2:
In the lower part of the page as shown in the next screenshot, fill the Group which you can consider as the base package. All other classes will be sub-package of this one.
Then add Artifact Id which will get copied to the Name field and the project will get created in this name.
Add a meaningful Description and select the Java version required. Please refer to the screenshot below for quick reference.
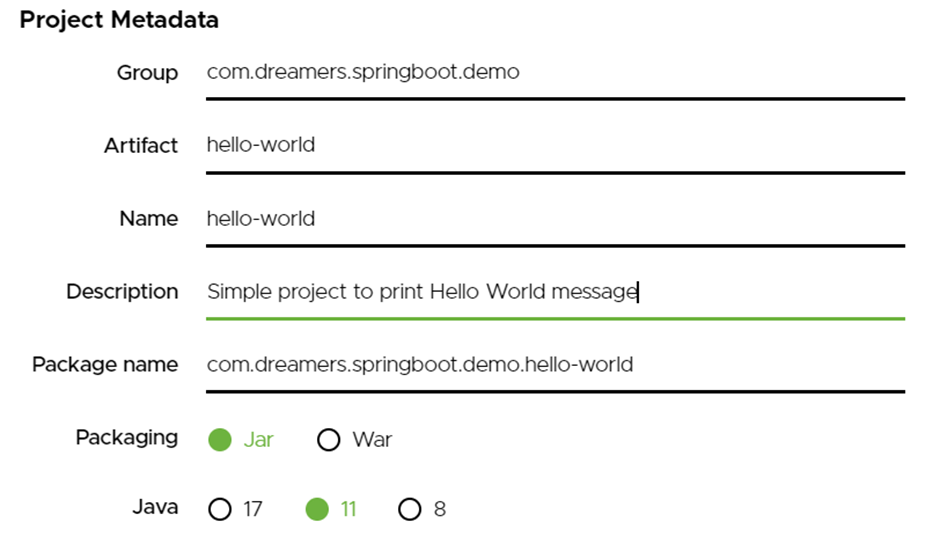
Now we will go to the dependency section, where we will select the dependencies to start with. Dependencies are project which have some pre-coded stuff which eases our life and reduce lot of setup work.
Step 3:
For this simple project, we need to add Spring-boot-starter-web dependency which brings with it lot of useful stuffs which we will discuss later.
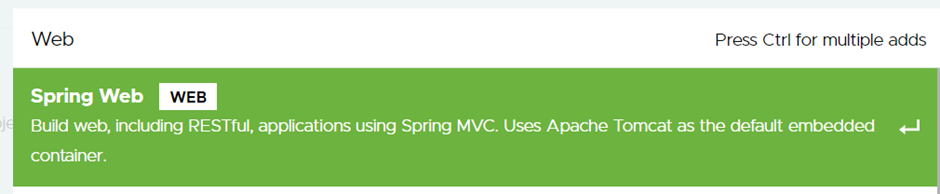
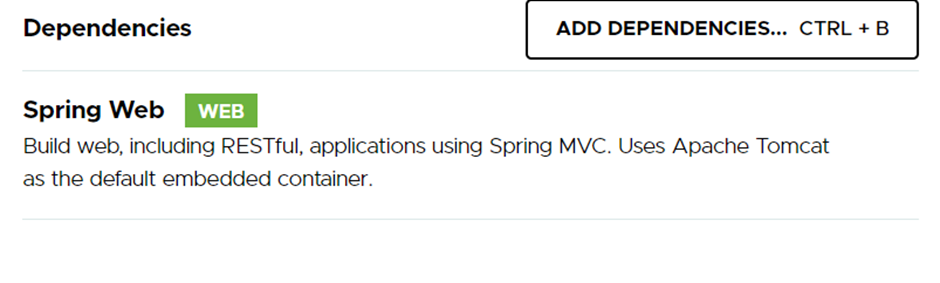
After this click on Generate button. You will get a zip downloaded which you can unzip in some folder and then use your preferred IDE like Eclipse or IntelliJ to work on.
In my example, I will use Spring Starter Suite (STS) which is easier to use.
Instead of following the above 3 steps and then downloading the zip, in STS, we will create project directly from the IDE as start.spring.io is plugged in with the IDE.
Alternative (on using STS):
You can download Spring Tool Suite 4 from Spring | Tools for your Operating System.
Once you un-jar the tool and run the .exe file, the IDE will open.
Like the screenshot below, you will get a Package Explorer, where you can click on the highlighted link. Alternatively, you can go to File -> New -> Spring Starter Project
After this click on Generate button. You will get a zip downloaded which you can unzip in some folder and then use your preferred IDE like Eclipse or IntelliJ to work on.
In my example, I will use Spring Starter Suite (STS) which is easier to use.
Instead of following the above 3 steps and then downloading the zip, in STS, we will create project directly from the IDE as start.spring.io is plugged in with the IDE.
Alternative (on using STS):
You can download Spring Tool Suite 4 from Spring | Tools for your Operating System.
Once you un-jar the tool and run the .exe file, the IDE will open.
Like the screenshot below, you will get a Package Explorer, where you can click on the highlighted link. Alternatively, you can go to File -> New -> Spring Starter Project
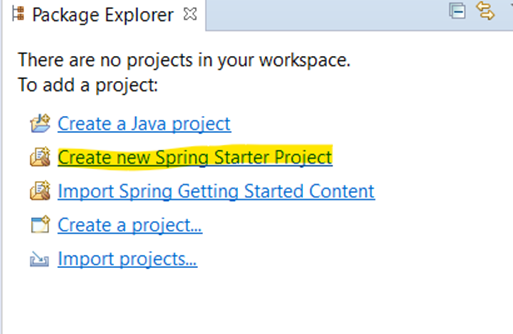
The following screen will open.
Fill the artifact, group, description and select the Java version and Maven as build tool
Let the project version remain as 0.0.1-SNAPSHOT for the time being. We can anyway change it anytime later and have to change as we update our code. SNAPSHOT – denotes that the version is in development and is yet to be released.
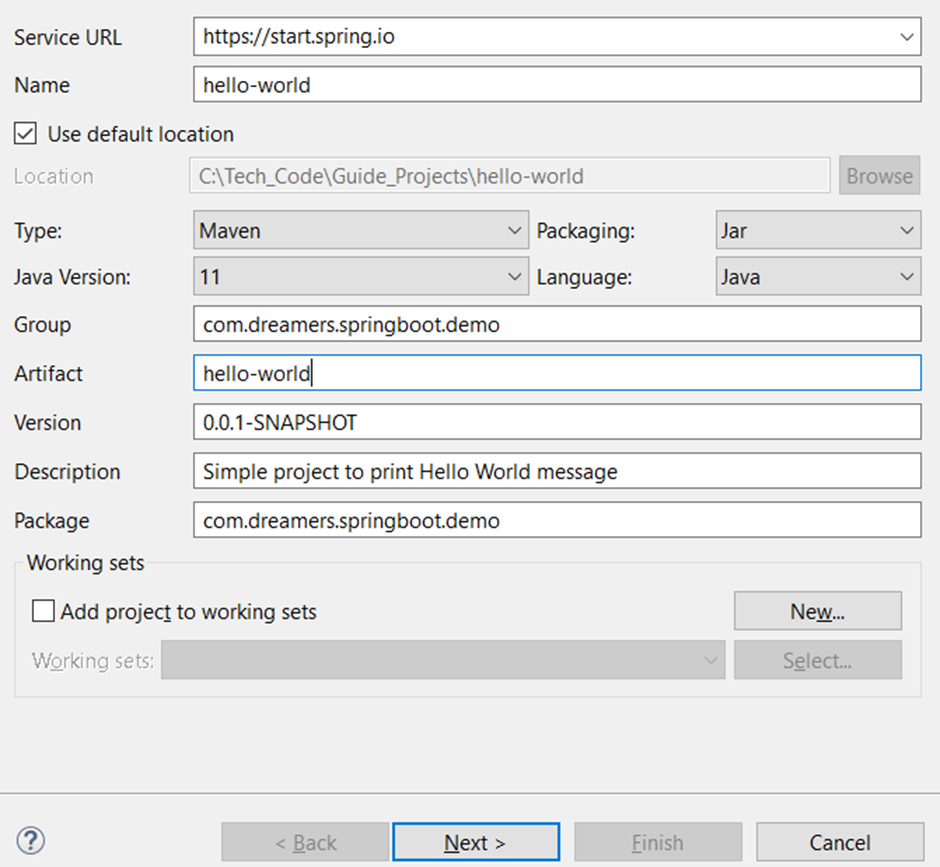
Click Next and add dependencies as before from the next screen. We are adding Spring Web dependency only for this project and click Finish button.
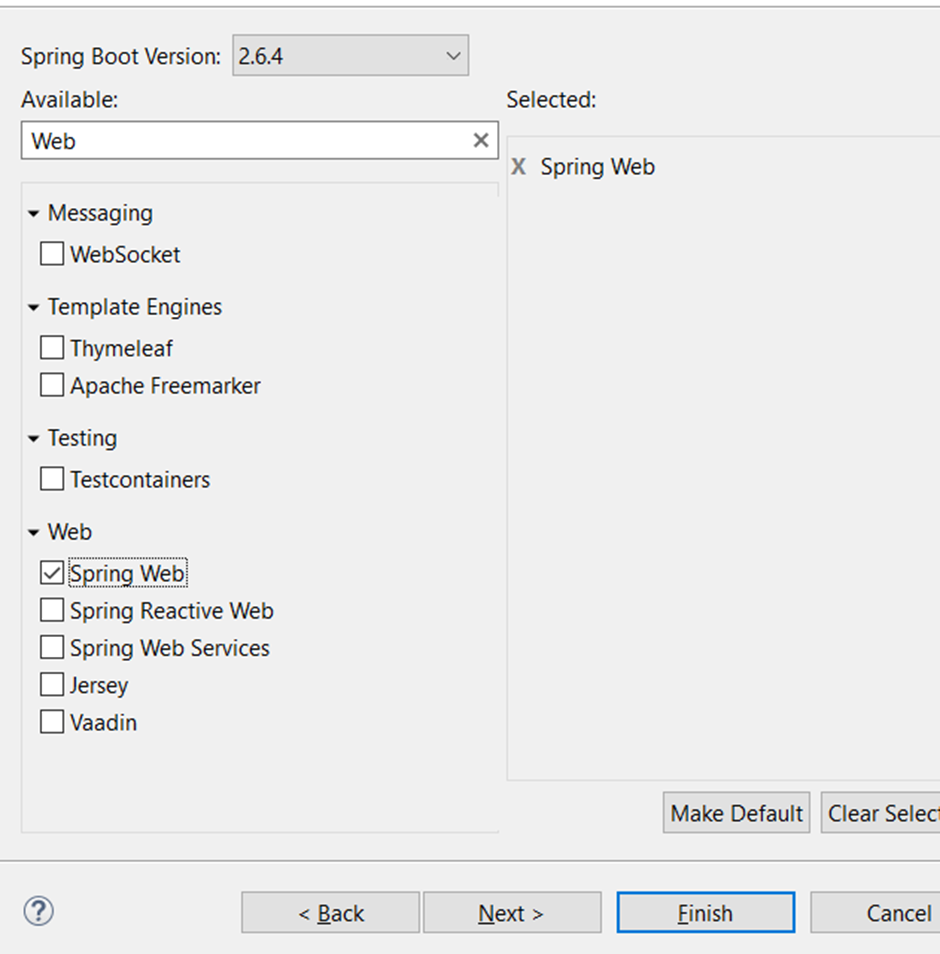
Step 4 :
Creation of project will take few minutes depending on your internet speed. At the bottom right section of the IDE, you can see a progress bar as shown in the screenshot below. This is because of the project added as dependency. IDE looks at the Maven configuration and downloads all the dependant projects. It is being downloaded and for the first time it takes few minutes.


Take a break and relax!!
Let’s diagnose the project
First let’s what all got created.
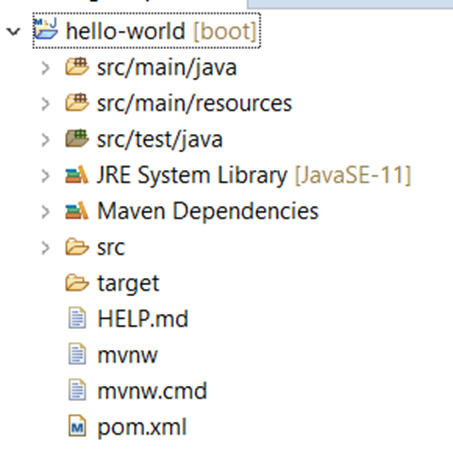
POM.XML
The last file is the most important file created which have all the configuration and dependency related information. It is the pom.xml. The full form of pom is Project Object Model. Let’s look at this file.
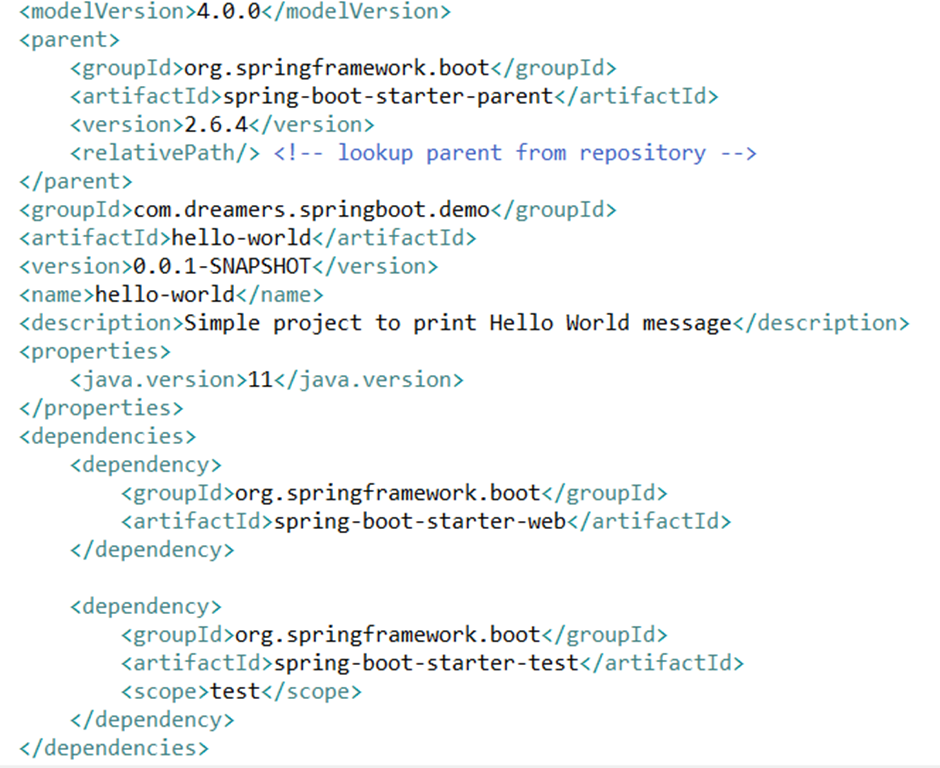
The details which we had filled during project creation are visible here.
Starter Parent
Apart from this, there is a parent tag under which spring-boot-starter-parent is mentioned. It is a base project which contains a number of Spring boot feature which our project will inherit and this is responsible for reducing the time of project creation from a day to few minutes. We will discuss it in detail later.
Dependencies
Then we can see 2 dependencies are added. The first one spring-boot-starter-web was the one we selected during creation of the project. It contains the basic functionalities required for developing a web application and the second one, spring-boot-starter-test is for creating unit tests which has a scope of test.
Maven Plugin
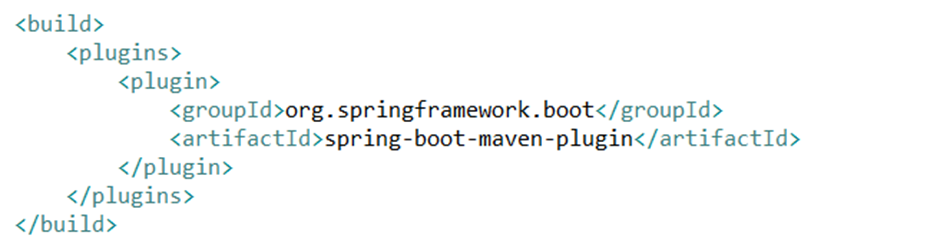
The spring-boot-maven-plugin helps in easily running spring boot applications. It also helps in building jar and war files from the application.
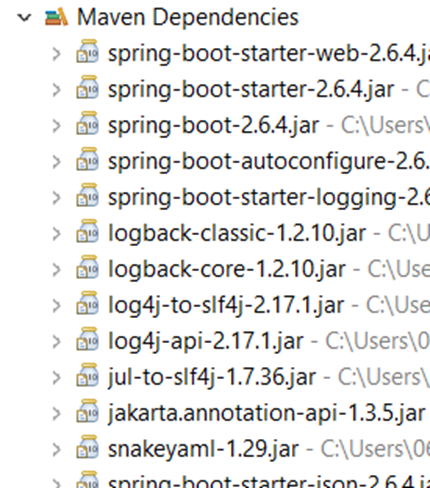
Now here we can see lot of JAR files under Maven Dependencies. Where are these coming from? This is actually the beauty of Maven. We do not have to search and down each of these. They have lot of codes which are required by our project to deploy the web application. But we are neither coding them nor do we download them one by one. These are downloaded by Maven based on the dependencies mentioned in POM.xml. But we only added 2 dependencies. Then how come we are having so many jars? These is because of transitive dependencies.
Transitive dependencies are those projects on which the projects on my dependency are dependent upon. Maven not only downloads the JAR files on which our project is directly dependent but also downloads the JARs of the transitive dependencies. If this is too complex to digest, then don’t worry. As we go on building our projects, we will get to see similar examples.
Spring boot application class
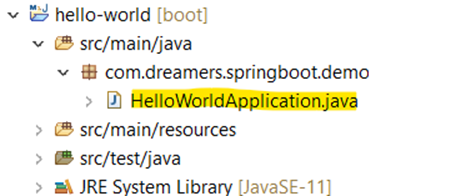
The class highlighted with yellow is the starting point of our application.
This class has two important things to discuss about
SpringBootApplication Annotation
This class is annotated with @SpringBootApplication. This annotation is one of the most important annotation of a Spring boot project. First it initializes spring framework and then Spring boot itself. Spring boot is running on top of spring framework and hence first initializes component scan of Spring framework and then it enables auto-configuration.
SpringApplication.run
As the class is annotated with @SpringBootApplication, on using SpringApplication.run with the classname, the application can be launched on a Tomcat server on a specific port just by running from boot dashboard. We will come to that step later.

We might have to add few more annotations in more complex project but we will come to that as we progress.
Test classes
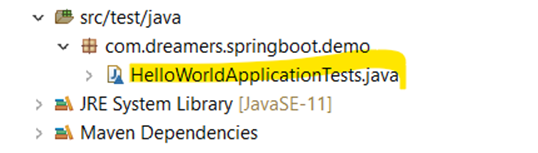
The .java files under src/test package are the Junit classes. We have to keep on adding more junit classes as we add new functionality in our class, if we want Junit to test our code.
Properties or YML file
Under src/main/resources, we have the application.properties file getting generated. This file contains the configuration like port, any specific configurable values or exception messages for running the project in local workspace. It can be renamed as application.yml as well when the key value syntax slightly changes.
Now let’s start the project.
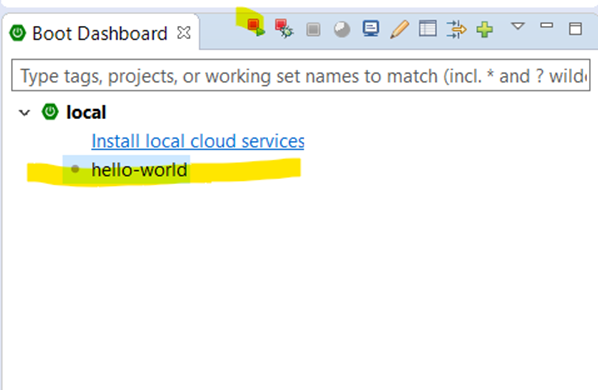
Go to the Boot dashboard in the lower left corner of the page. If it is not visible then add it from Menu : Window -> Show View -> Other
Click on the start button highlighted after selecting the hello-world. You will see that in the log you will get a message saying – Started HelloWorldApplication in xxx seconds
So, the tomcat server is up and we succeeded in creating a Spring boot application in few minutes. However, this one is not having any custom code yet. Now we have to customize the code as per our requirement in our next step
Conclusion
In this section, we learnt –
- How to create a spring boot project?
- Some basic dependencies
- What is POM.xml and what does it contain?
- Project hierarchy
- How to start the server?
2 thoughts on “How to create a simple spring boot application?”